A bookmark identifies a location or a section of text in a document that you can refer to at a later time. In this article, I am going to introduce how to add bookmarks to paragraphs or selected range of text in an existing Word document, and how to delete a bookmark by its index using Free Spire.Doc for Java.
Installing Spire.Doc.jar
If you create a Maven project, you can easily import the jar in your application using the following configurations. For non-Maven projects, download the jar file from this link and add it as a dependency in your application.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>http://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId> e-iceblue </groupId>
<artifactId>spire.doc.free</artifactId>
<version>3.9.0</version>
</dependency>
</dependencies>
Example 1. Add a Bookmark to a Paragraph
import com.spire.doc.*;
import com.spire.doc.documents.Paragraph;
public class AddBookmarkToParagraph {
public static void main(String[] args) {
//Create a Document object
Document doc = new
Document();
//Load a sample Word file
doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.docx");
//Get the second paragraph
Paragraph paragraph = doc.getSections().get(0).getParagraphs().get(1);
//Create a bookmark start
BookmarkStart start = paragraph.appendBookmarkStart("myBookmark");
//Insert it at the beginning of the paragraph
paragraph.getItems().insert(0,start);
//Append a bookmark end at the end of the paragraph
paragraph.appendBookmarkEnd("myBookmark");
//save the file
doc.saveToFile("output/AddBookmarkToParagraph.docx", FileFormat.Docx_2013);
}
}
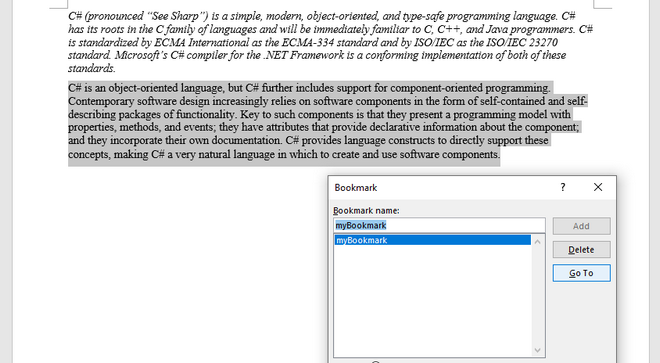
Example 2. Add a Bookmark to Selected Text
import com.spire.doc.BookmarkEnd;
import com.spire.doc.BookmarkStart;
import com.spire.doc.Document;
import com.spire.doc.FileFormat;
import com.spire.doc.documents.Paragraph;
import com.spire.doc.documents.TextSelection;
public class AddBookmarkToSelectedRangeOfText {
public static void main(String[] args) {
//Create a Document object
Document doc = new
Document();
//Load a sample Word file
doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.docx");
//String to find
String stringToFind = "C# is
standardized by ECMA International as the ECMA-334 standard and by ISO/IEC as the ISO/IEC 23270 standard.";
//Find the selected text from the document
TextSelection[] finds = doc.findAllString(stringToFind, false, true);
TextSelection specificText = finds[0];
//Find the paragraph where the text is located
Paragraph para = specificText.getAsOneRange().getOwnerParagraph();
//Get the index of the text in the paragraph
int index =
para.getChildObjects().indexOf(specificText.getAsOneRange());
//Create a bookmark start
BookmarkStart start = para.appendBookmarkStart("myBookmark");
//Insert the bookmark start at the index position
para.getChildObjects().insert(index, start);
//Create a bookmark end
BookmarkEnd end = para.appendBookmarkEnd("myBookmark");
//Insert the bookmark end at the end of the selected text
para.getChildObjects().insert(index + 2, end);
//Save the document to another file
doc.saveToFile("output/AddBookmarkToSelectedText.docx", FileFormat.Docx_2013);
}
}
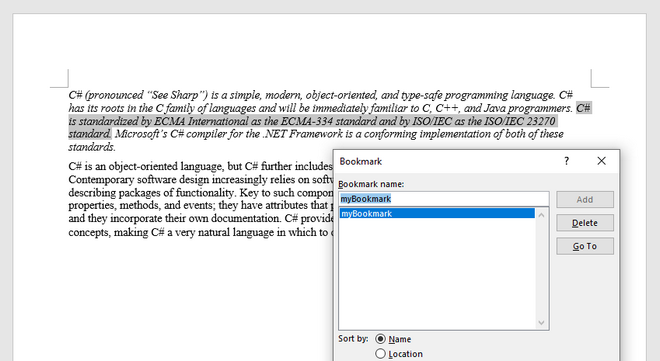
Example 3. Delete a Bookmark from a Word Document
import com.spire.doc.Bookmark;
import com.spire.doc.Document;
import com.spire.doc.FileFormat;
public class DeleteBookmark {
public static void main(String[] args) {
//Create a Document object
Document doc = new
Document();
//Load an existing Word file
doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\Bookmarks.docx");
//Get bookmark by its index
Bookmark bookmark = doc.getBookmarks().get(0);
//Remove the bookmark
doc.getBookmarks().remove(bookmark);
//Save the document.
doc.saveToFile("output/RemoveBookmark.docx", FileFormat.Docx);
}
}
Write a comment