Although PDF is a perfect document format, sometimes we still need to convert it to image format, because images can be displayed directly on most devices, while PDF needs a special reader. In this article, you’ll learn how to programmatically convert PDF to popular image formats like JPG, PNG and SVG by using Spire.PDF for Java.
Installing Spire.Pdf.jar
If you create a Maven project, you can easily import the jar in your application using the following configurations. For non-Maven projects, download the jar file from this link and add it as a dependency in your application.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>http://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId> e-iceblue</groupId>
<artifactId>spire.pdf</artifactId>
<verson>4.12.1</version>
</dependency>
</dependencies>
Example 1. Convert PDF to JPG
import com.spire.pdf.PdfDocument;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
public class ConvertPdfToJpg {
public static void main(String[] args)
throws Exception {
//Create a PdfDocument object
PdfDocument doc = new
PdfDocument();
//Load a sample PDF document
doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.pdf");
//Declare a BufferedImage variable
BufferedImage image;
//Loop through the pages
for (int i = 0; i < doc.getPages().getCount(); i++) {
//Save the current page as a buffered image
image = doc.saveAsImage(i);
//Write the image data as a .jpg file
File file = new File("C:\\Users\\Administrator\\Desktop\\Output\\" + String.format("ToImage-img-%d.jpg", i));
ImageIO.write(image, "JPG", file);
}
doc.close();
}
}
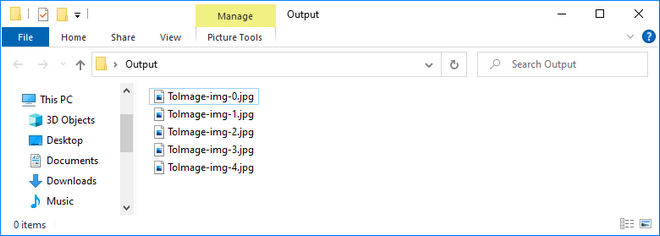
Example 2. Convert PDF to Transparent PNG
import com.spire.pdf.*;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
public class ConvertPdfToTransparentPng {
public static void main(String[] args) throws Exception{
//Create a PdfDocument object
PdfDocument doc = new PdfDocument();
//Load a sample PDF document
doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.pdf");
//Specify the background transparent value of the converted image as 0
doc.getConvertOptions().setPdfToImageOptions(0);
//Declare a BufferedImage variable
BufferedImage image;
//Loop through the pages
for (int i = 0; i < doc.getPages().getCount(); i++) {
//Save the current page as a buffered image
image = doc.saveAsImage(i);
//Write the image data as a .png file
File file = new File("C:\\Users\\Administrator\\Desktop\\Output\\" + String.format("ToImage-%d.png", i));
ImageIO.write(image, "png", file);
}
doc.close();
}
}
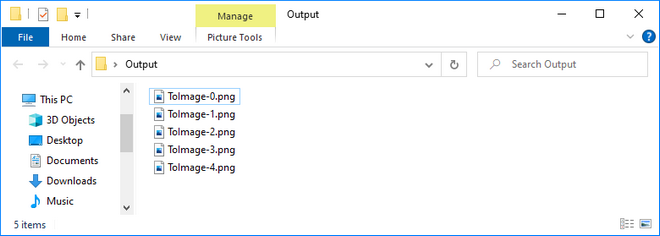
Example 3. Convert PDF to SVG
import com.spire.pdf.FileFormat;
import com.spire.pdf.PdfDocument;
public class ConvertPdfToSvg {
public static void main(String[] args) {
//Create a PdfDocument object
PdfDocument doc = new
PdfDocument();
//Load a sample PDF document
doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.pdf");
//If you want the PDF document to be converted as a single SVG file, pass true to
setOutputToOneSvg() method
//doc.getConvertOptions().setOutputToOneSvg(true);
//Save each page as an individual SVG file
doc.saveToFile("C:\\Users\\Administrator\\Desktop\\Output\\ToSVG.svg", FileFormat.SVG);
doc.close();
}
}
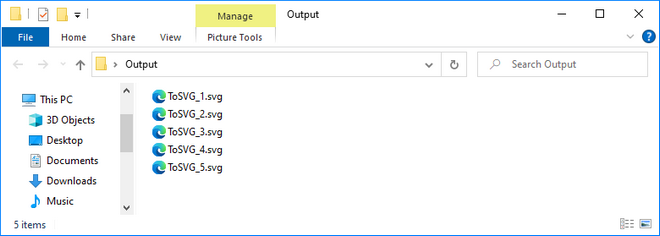
Write a comment