Tables can be used to organize content and present it in an organized way. By using tables, your audience can see how the content in one cell relates to that in another cell. In this article, I am going introduce how to programmatically create a table in a PowerPoint presentation, and how to merge or split table cells by using Spire.Presentation for Java.
Installing Spire.Presentation.jar
If you use Maven, you can easily import the Spire.Presentation.jar in your application using the following configurations. For non-Maven projects, please download the jar file from this link and add it as a dependency in your program.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>https://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId> e-iceblue </groupId>
<artifactId>spire.presentation</artifactId>
<version>4.10.1</version>
</dependency>
</dependencies>
Example 1. Create a Table and Apply a Built-in Table Style
import com.spire.presentation.*;
public class AddTable {
public static void main(String[] args)
throws Exception {
//Create a Presentation instance
Presentation presentation = new Presentation();
presentation.getSlideSize().setType(SlideSizeType.SCREEN_16_X_9);
//Define column widths and row heights
Double[] widths = new
Double[]{100d, 100d, 100d, 150d, 100d, 100d};
Double[] heights = new Double[]{15d, 15d, 15d, 15d, 15d};
//Add a table
ITable table = presentation.getSlides().get(0).getShapes().appendTable(20, 70, widths, heights);
//Define table data
String[][] data = new
String[][]{
{"Emp#", "Name", "Job", "HiringD", "Salary", "Dept#"},
{"7369", "Andrews", "Engineer", "05/12/2006", "1600.00", "20"},
{"7499", "Nero", "Technician", "03/23/2006", "1800.00", "30"},
{"7521", "Adams", "Technician", "11/03/2007", "2400.00", "30"},
{"7566", "Martin", "Manager", "12/21/2007", "2600.00", "10"},
};
//Loop through the row and column of the table
for (int i = 0; i < data.length; i++) {
for (int j = 0; j < data[i].length; j++) {
//Fill the table with data
table.get(j,
i).getTextFrame().setText(data[i][j]);
//Align text in each cell to
center
table.get(j,
i).getTextFrame().getParagraphs().get(0).setAlignment(TextAlignmentType.CENTER);
}
}
//Apply a built-in style to table
table.setStylePreset(TableStylePreset.LIGHT_STYLE_1_ACCENT_1);
//Save the document
presentation.saveToFile("output/AddTable.pptx", FileFormat.PPTX_2013);
}
}
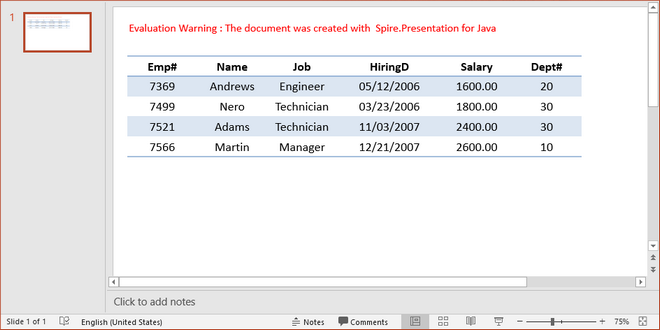
Example 2. Merge and Split Table Cells
import com.spire.presentation.*;
public class SplitOrMergeCell {
public static void main(String[] args)
throws Exception {
//Create a Presentation instance
Presentation presentation = new Presentation();
presentation.getSlideSize().setType(SlideSizeType.SCREEN_16_X_9);
//Define column widths and row heights
Double[] widths = new
Double[]{130d, 130d, 130d, 130d, 130d};
Double[] heights = new Double[]{30d, 30d, 30d, 30d, 30d};
//Add a table
ITable table = presentation.getSlides().get(0).getShapes().appendTable(20, 70, widths, heights);
table.setStylePreset(TableStylePreset.NO_STYLE_TABLE_GRID);
//Insert text to cells
table.get(0,0).getTextFrame().setText("First Name");
table.get(2,0).getTextFrame().setText("Last
Name");
table.get(4,0).getTextFrame().setText("Photo");
table.get(0,1).getTextFrame().setText("Birth
Date");
table.get(2,1).getTextFrame().setText("Email
Address");
table.get(0,2).getTextFrame().setText("Mobile");
table.get(2,2).getTextFrame().setText("Home");
table.get(0,3).getTextFrame().setText("Address");
table.get(0,4).getTextFrame().setText("Additional
Info");
//Merge the neighbor cells
table.mergeCells(table.get(4,0),table.get(4,4),false );
table.mergeCells(table.get(1,3),table.get(3,3),false);
table.mergeCells(table.get(1,4),table.get(3,4),false);
//Split a cell into 3 columns
table.get(1,1).split(1,3);
//Save the document to file
presentation.saveToFile("output/SplitAndMergeCells.pptx", FileFormat.PPTX_2013);
}
}
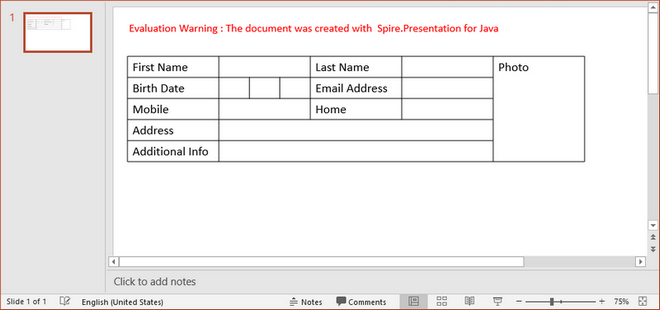
Example 3. Some Other Operations
· Access an existing table
Presentation presentation = new Presentation();
presentation.loadFromFile("Table.pptx");
ITable table = null;
for (Object shape: presentation.getSlides().get(0).getShapes()
) {
if (shape instanceof ITable){
table = (ITable)shape;
}
}
· Add a row or a column
//add a row by duplicating the last row
table.getTableRows().append(table.getTableRows().get(table.getTableRows().getCount()-1));
//obtain the new
TableRow lastRow = table.getTableRows().get(table.getTableRows().getCount()-1);
//add a column by duplicating the last column
table.getColumnsList().add(table.getColumnsList().get(table.getColumnsList().getCount()-1));
//obtain the new column added
TableColumn lastColumn = table.getColumnsList().get(table.getColumnsList().getCount()-1);
· Insert a specific row or column to the specified position
table.getTableRows().insert(0, table.getTableRows().get(0));
table.getColumnsList().insert(0, table.getColumnsList().get(0));
· Set the row height or column width
table.getTableRows().get(0).setHeight(50);
table.getColumnsList().get(0).setWidth(100);
· Delete a row or a column
table.getTableRows().removeAt(0, false);
table.getColumnsList().removeAt(0, false);
· Fill a cell with picture
table.get(0,0).getFillFormat().setFillType(FillFormatType.PICTURE);
table.get(0,0).getFillFormat().getPictureFill().getPicture().setUrl((new java.io.File("bkg.jpg")).getAbsolutePath());
· Fill a cell with solid color
table.get(0,1).getFillFormat().setFillType(FillFormatType.SOLID);
table.get(0,1).getFillFormat().getSolidColor().setColor(Color.blue);
· Set border color
table.setTableBorder(TableBorderType.All, 1, Color.black);
· Set the text alignment within a cell
table.get(0,0).getTextFrame().getParagraphs().get(0).setAlignment(TextAlignmentType.CENTER);
· Set the font name and font size of text in a cell
table.get(0,0).getTextFrame().getParagraphs().get(0).getTextRanges().get(0).setLatinFont(new TextFont("Calibri"));
table.get(0,0).getTextFrame().getParagraphs().get(0).getTextRanges().get(0).setFontHeight(20f);
· Set the text color
table.get(0,0).getTextFrame().getParagraphs().get(0).getTextRanges().get(0).getFill().setFillType(FillFormatType.SOLID);
table.get(0,0).getTextFrame().getParagraphs().get(0).getTextRanges().get(0).getFill().getSolidColor().setColor(color.red);
Write a comment