When you receive a Word document, you may want to modify the font styles of the specific paragraphs in order to achieve a standardized or aesthetic requirement. In this article, I am going to introduce how to programmatically change font name, font size, font color and font style (bold, italic) by using Spire.Doc for Java.
Installing Spire.Doc.jar
If you create a Maven project, you can easily import the jar in your application using the following configurations. For non-Maven projects, download the jar file from this link and add it as a dependency in your application.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>http://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId> e-iceblue</groupId>
<artifactId>spire.doc</artifactId>
<version>4.6.2</version>
</dependency>
</dependencies>
Using the code
import com.spire.doc.Document;
import com.spire.doc.FileFormat;
import com.spire.doc.Section;
import com.spire.doc.documents.Paragraph;
import com.spire.doc.fields.TextRange;
import java.awt.*;
public class ChangeFontStyles {
public static void main(String[] args) {
//Create a Document instance
Document doc = new
Document();
//Load the Word document
doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.docx");
//Get the first section and second paragraph
Section section = doc.getSections().get(0);
//Get the first paragraph
Paragraph paragraphOne = section.getParagraphs().get(0);
//Iterate through the childObjects of the first paragraph
for (int i = 0; i < paragraphOne.getChildObjects().getCount(); i ++)
{
//Change font color of the text
if (
paragraphOne.getChildObjects().get(i) instanceof TextRange)
{
TextRange tr = (TextRange) paragraphOne.getChildObjects().get(i);
tr.getCharacterFormat().setTextColor(Color.green);
}
}
//Get the second paragraph
Paragraph paragraphTwo = section.getParagraphs().get(1);
//Iterate through the childObjects of the second paragraph
for (int i = 0; i < paragraphTwo.getChildObjects().getCount(); i ++)
{
//Change font size of the text
if (
paragraphOne.getChildObjects().get(i) instanceof TextRange)
{
TextRange tr = (TextRange) paragraphTwo.getChildObjects().get(i);
tr.getCharacterFormat().setFontSize(16f);
}
}
//Get the third paragraph
Paragraph paragraphThree= section.getParagraphs().get(2);
//Iterate through the childObjects of the third paragraph
for (int i = 0; i < paragraphThree.getChildObjects().getCount(); i ++)
{
//Change the font name and make the text bold &
italic
if (
paragraphOne.getChildObjects().get(i) instanceof TextRange)
{
TextRange tr = (TextRange) paragraphThree.getChildObjects().get(i);
tr.getCharacterFormat().setFontName("Times New Roman");
tr.getCharacterFormat().setBold(true);
tr.getCharacterFormat().setItalic(true);
}
}
//Save the document
doc.saveToFile("output/ChangeFontStyles.docx", FileFormat.Docx_2013);
}
}
Output
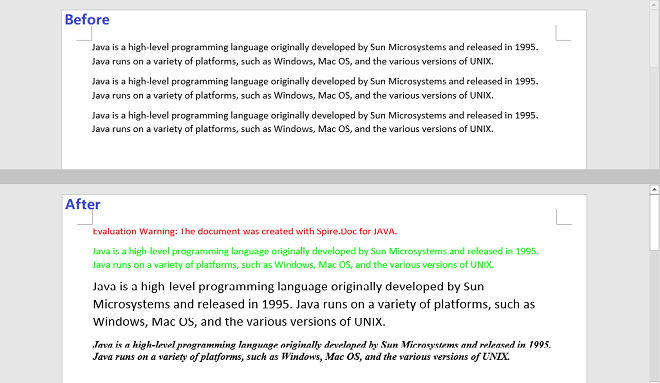
Write a comment