PDF layers allow documents to have content placed either above or below other content within the document. Individual layers within a PDF can be displayed or hidden by the user if needed. In this article, I am going to introduce how to add, hide or delete layers in a PDF document by using Spire.PDF for Java.
Below is a screenshot of the sample PDF file.
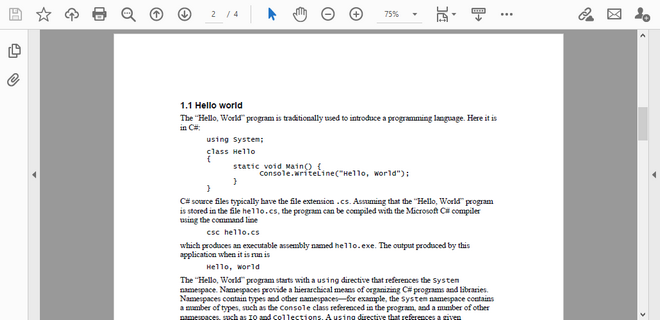
Installing Spire.Pdf.jar
If you create a Maven project, you can easily import the jar in your application using the following configurations. For non-Maven projects, download the jar file from this link and add it as a dependency in your application.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>http://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId> e-iceblue</groupId>
<artifactId>spire.pdf</artifactId>
<verson>4.1.2</version>
</dependency>
</dependencies>
Example 1. Add layers to PDF
import com.spire.pdf.PdfDocument;
import com.spire.pdf.PdfPageBase;
import com.spire.pdf.graphics.*;
import com.spire.pdf.graphics.layer.PdfLayer;
import java.awt.*;
import java.awt.geom.Dimension2D;
import java.io.IOException;
public class AddLayersToPdf {
public static void main(String[] args)
throws IOException {
//Create a PdfDocument object and load the sample PDF file
PdfDocument pdf = new
PdfDocument();
pdf.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.pdf");
//Invoke AddLayerWatermark method to add a watermark layer
AddLayerWatermark(pdf);
//Invoke AddLayerHeader method to add a header layer
AddLayerHeader(pdf);
//Save to file
pdf.saveToFile("AddLayers.pdf");
pdf.close();
}
private static void AddLayerWatermark(PdfDocument
doc) throws IOException {
//Create a layer named "watermark"
PdfLayer layer = doc.getLayers().addLayer("watermark");
//Create a font
PdfTrueTypeFont font = new PdfTrueTypeFont(new
Font("Arial",
Font.PLAIN,36),true);
//Specify watermark text
String watermarkText = "Internal
use only";
//Get text size
Dimension2D fontSize = font.measureString(watermarkText);
//Calculate two offsets
float offset1 =
(float)(fontSize.getWidth() * Math.sqrt(2) / 4);
float offset2 =
(float)(fontSize.getHeight() * Math.sqrt(2) / 4);
//Get page count
int pageCount =
doc.getPages().getCount();
//Declare two variables
PdfPageBase page;
PdfCanvas canvas;
//Loop through the pages
for (int i = 0; i < pageCount; i++) {
page = doc.getPages().get(i);
//Create a canvas from layer
canvas = layer.createGraphics(page.getCanvas());
canvas.translateTransform(canvas.getSize().getWidth() / 2
- offset1 - offset2, canvas.getSize().getHeight() / 2 + offset1 - offset2);
canvas.setTransparency(0.4f);
canvas.rotateTransform(-45);
//Draw sting on the canvas of layer
canvas.drawString(watermarkText, font,
PdfBrushes.getDarkRed(), 0, 0);
}
}
private static void AddLayerHeader(PdfDocument doc)
{
//Create a layer named "header"
PdfLayer layer = doc.getLayers().addLayer("header");
//Get page size
Dimension2D size = doc.getPages().get(0).getSize();
//Specify the initial values of X and y
float x = 90;
float y = 10;
//Get page count
int pageCount =
doc.getPages().getCount();
//Declare two variables
PdfPageBase page;
PdfCanvas canvas;
//Loop through the pages
for (int i = 0; i < pageCount; i++) {
//Draw an image on the layer
PdfImage pdfImage = PdfImage.fromFile("C:\\Users\\Administrator\\Desktop\\logo2.png");
float width = pdfImage.getWidth();
float height = pdfImage.getHeight();
page = doc.getPages().get(i);
canvas = layer.createGraphics(page.getCanvas());
canvas.drawImage(pdfImage, x, y, width, height);
//Draw a line on the layer
PdfPen pen = new PdfPen(PdfBrushes.getDarkGray(), 3f);
canvas.drawLine(pen, x, y + height + 5, size.getWidth() - x, y + height + 2);
}
}
}
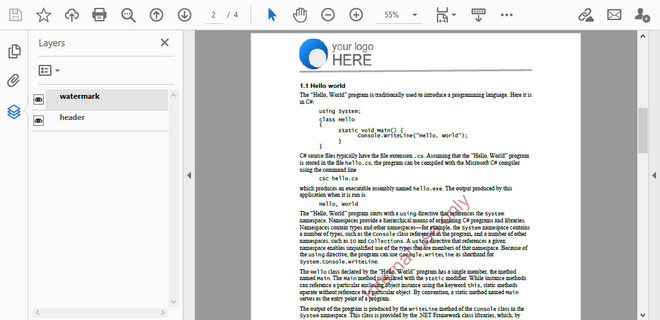
Example 2. Set visibility of layer in PDF
import com.spire.pdf.FileFormat;
import com.spire.pdf.PdfDocument;
import com.spire.pdf.graphics.layer.PdfVisibility;
public class SetLayerVisibility {
public static void main(String[] args) {
//Create a PdfDocument object and load the sample PDF file
PdfDocument pdf = new
PdfDocument();
pdf.loadFromFile("C:\\Users\\Administrator\\Desktop\\Layers.pdf");
//Set the visibility of the first layer to off
pdf.getLayers().get(0).setVisibility(PdfVisibility.Off);
//Save to file
pdf.saveToFile("HideLayer.pdf", FileFormat.PDF);
pdf.dispose();
}
}
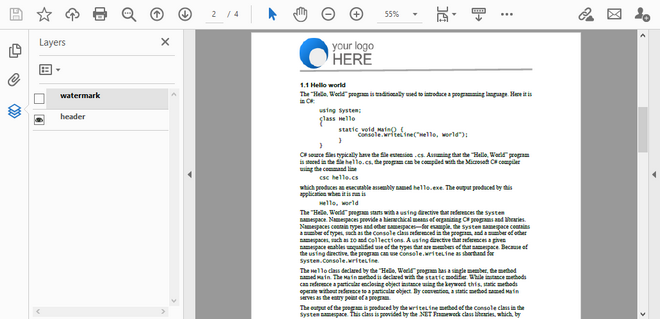
Example 3. Delete layers from PDF
import com.spire.pdf.PdfDocument;
public class DeleteLayers {
public static void main(String[] args) {
//Create a PdfDocument
object and load the sample PDF file
PdfDocument pdf = new PdfDocument();
pdf.loadFromFile("C:\\Users\\Administrator\\Desktop\\Layers.pdf");
//Delete the specific
layer by its name
pdf.getLayers().removeLayer("watermark");
//Save to file
pdf.saveToFile("DeleteLayer.pdf");
pdf.close();
}
}
Write a comment