Sometimes, you may want to export Excel data to Word table without embedding the whole worksheet into the Word document. You can copy (Ctrl + C) the cells of a selected range and paste (Ctrl + V) them into Word as a table with or without the source formatting. In this article, I am going to introduce how to programmatically convert Excel data to Word table maintaining cell formatting and font styles, using Spire.Office for Java.
Installing Spire.Office.jar
If you create a Maven project, you can easily import the jar in your application using the following configurations. For non-Maven projects, download the jar file from this link and add it as a dependency in your application.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>http://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId> e-iceblue</groupId>
<artifactId>spire.office</artifactId>
<version>4.4.6</version>
</dependency>
</dependencies>
Using the code
import com.spire.doc.Document;
import com.spire.doc.FileFormat;
import com.spire.doc.Table;
import com.spire.doc.TableCell;
import com.spire.doc.documents.HorizontalAlignment;
import com.spire.doc.fields.TextRange;
import com.spire.xls.CellRange;
import com.spire.xls.Workbook;
import com.spire.xls.Worksheet;
public class ExportDataToWord {
public static void main(String[] args) {
//Create a Workbook object and load the sample excel file
Workbook workbook = new
Workbook();
workbook.loadFromFile("C:\\Users\\Administrator\\Desktop\\Data.xlsx");
//Get the first worksheet
Worksheet sheet = workbook.getWorksheets().get(0);
//Create a word document
Document doc = new
Document();
//Add a table to Word
Table table = doc.addSection().addTable(true);
//Set row and column number of table
table.resetCells(sheet.getLastRow(), sheet.getLastColumn());
for (int r = 1; r <= sheet.getLastRow(); r++)
{
for (int c = 1; c <= sheet.getLastColumn(); c++)
{
CellRange xCell = sheet.getCellRange(r,c);
TableCell wCell = table.getRows().get(r-1).getCells().get(c-1);
//Fill the word table with data from
excel
TextRange textRange =
wCell.addParagraph().appendText(xCell.getNumberText());
//Copy font and cell style from excel
to word
CopyStyle(textRange, xCell, wCell);
}
}
//Set column width of word table
for (int i = 0; i < table.getRows().getCount(); i++)
{
for (int j = 0; j < table.getRows().get(i).getCells().getCount(); j++)
{
table.getRows().get(i).getCells().get(j).setWidth(60f);
}
}
//Save to file
doc.saveToFile("output/Result.docx", FileFormat.Docx);
}
private static void CopyStyle(TextRange wTextRange,
CellRange xCell, TableCell wCell)
{
//Copy font style
wTextRange.getCharacterFormat().setTextColor(xCell.getStyle().getFont().getColor());
wTextRange.getCharacterFormat().setFontSize((float)xCell.getStyle().getFont().getSize());
wTextRange.getCharacterFormat().setFontName(xCell.getStyle().getFont().getFontName());
wTextRange.getCharacterFormat().setBold(xCell.getStyle().getFont().isBold());
wTextRange.getCharacterFormat().setItalic(xCell.getStyle().getFont().isItalic());
//Copy backcolor
wCell.getCellFormat().setBackColor(xCell.getStyle().getColor());
//Copy horizontal alignment
switch (xCell.getHorizontalAlignment())
{
case Left:
wTextRange.getOwnerParagraph().getFormat().setHorizontalAlignment(HorizontalAlignment.Left);
break;
case Center:
wTextRange.getOwnerParagraph().getFormat().setHorizontalAlignment(HorizontalAlignment.Center);
break;
case Right:
wTextRange.getOwnerParagraph().getFormat().setHorizontalAlignment(HorizontalAlignment.Right);
break;
default:
break;
}
}
}
Output
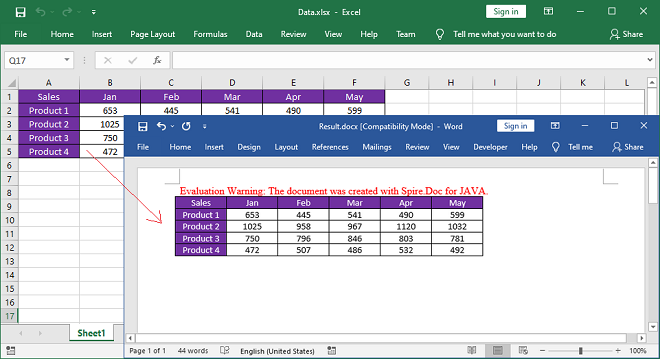
Write a comment