In this article, I am going to introduce how to convert an URL (or a web page) and HTML strings to PDF by using Free Spire.PDF for Java with QT plugin. The plugin is an open-source library and be downloaded from the following links. Download the package that fits in with your operation system, and unzip it somewhere on your hard drive.
Installing Spire.Pdf.jar
If you create a Maven project, you can easily import the jar in your application using the following configurations. For non-Maven projects, download the jar file from this link and add it as a dependency in your application.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>http://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId> e-iceblue </groupId>
<artifactId>spire.pdf</artifactId>
<verson>4.1.2</version>
</dependency>
</dependencies>
Example 1. Convert URL to PDF
import com.spire.pdf.graphics.PdfMargins;
import com.spire.pdf.htmlconverter.qt.HtmlConverter;
import com.spire.pdf.htmlconverter.qt.Size;
public class ConvertUrlToPdf {
public static void main(String[] args)
{
//Specify the url path
String url =
"https://www.stackoverflow.com/";
//Specify the output file path
String fileName = "output/Result.pdf";
//Specify the plugin path
String pluginPath = "E:\\Products\\Java\\plugins-windows-x64";
//Set plugin path
HtmlConverter.setPluginPath(pluginPath);
//Convert URL to PDF
HtmlConverter.convert(url, fileName,
true, 1000000, new
Size(1000f, 900f),
new PdfMargins(0));
}
}
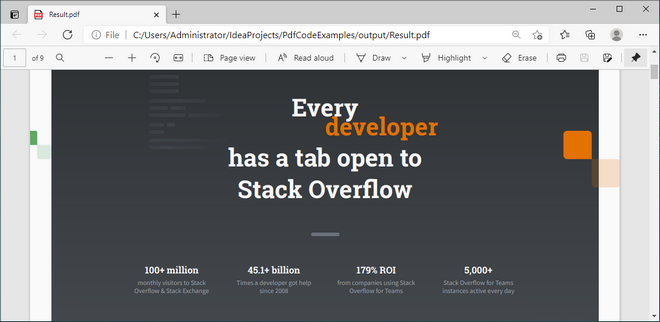
Example 2. Convert HTML string to PDF
import com.spire.pdf.graphics.PdfMargins;
import com.spire.pdf.htmlconverter.LoadHtmlType;
import com.spire.pdf.htmlconverter.qt.HtmlConverter;
import com.spire.pdf.htmlconverter.qt.Size;
public class ConvertHtmlStringToPdf {
public static void main(String[] args)
{
//Specify the html string
String htmlString = "<html>" +
"<body>" +
"<h2>An Unordered HTML
List</h2>\n" +
"<ul>" +
"
<li>Coffee</li>" +
"
<li>Tea</li>" +
"
<li>Milk</li>" +
"</ul>" +
"<h2>An Ordered HTML
List</h2>" +
"<ol>" +
"
<li>Coffee</li>" +
"
<li>Tea</li>" +
"
<li>Milk</li>" +
"</ol>" +
"</body>" +
"</html>";
//Specify the output file path
String outputFile = "output/HtmlToPdf.pdf";
//Specify the plugin path
String pluginPath = "E:\\Products\\Java\\plugins-windows-x64";
//Set plugin path
HtmlConverter.setPluginPath(pluginPath);
//Convert html string to PDF
HtmlConverter.convert(htmlString, outputFile, true,
100000, new Size(600, 900), new
PdfMargins(0), LoadHtmlType.Source_Code);
}
}
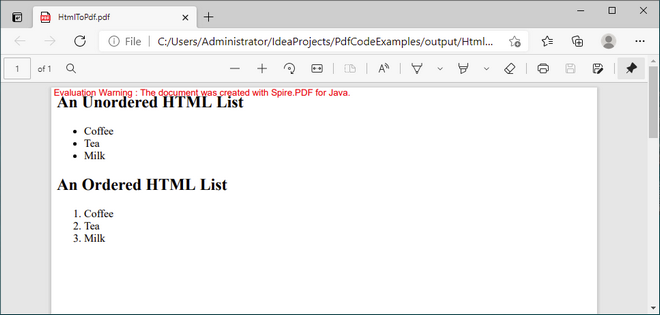
Write a comment