Mail merge in Microsoft Word allows you to send personalized letters and emails without having to customize each letter. In this article, I am going to show you how to create a template by inserting merge fields in it, and how to merge text values and image into merge fields, by using Free Spire.Doc for Java.
Installing Spire.Doc.jar
If you create a Maven project, you can easily import the jar in your application using the following configurations. For non-Maven projects, download the jar file from this link and add it as a dependency in your application.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>http://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId> e-iceblue </groupId>
<artifactId>spire.doc.free</artifactId>
<version>2.7.3</version>
</dependency>
</dependencies>
Example 1. Create merge fields
import com.spire.doc.Document;
import com.spire.doc.FieldType;
import com.spire.doc.FileFormat;
import com.spire.doc.Section;
import
com.spire.doc.documents.BreakType;
import com.spire.doc.documents.Paragraph;
public class CreateMailMerge {
public static void main(String[] args) {
//Create a Document object
Document document = new
Document();
//Add a section
Section section = document.addSection();
//Add a paragraph
Paragraph paragraph = section.addParagraph();
//Add text and mail merge fields to the paragraph
paragraph.appendText("Name:
");
paragraph.appendField("Name",
FieldType.Field_Merge_Field);
paragraph.appendBreak(BreakType.Line_Break);
paragraph.appendText("Phone: ");
paragraph.appendField("Phone",
FieldType.Field_Merge_Field);
paragraph.appendBreak(BreakType.Line_Break);
paragraph.appendText("Date: ");
paragraph.appendField("Date",
FieldType.Field_Merge_Field);
paragraph.appendBreak(BreakType.Line_Break);
paragraph.appendText("Portrait: ");
paragraph.appendField("Image:Portrait",
FieldType.Field_Merge_Field);
//Save the document
document.saveToFile("Template.docx", FileFormat.Docx_2013);
}
}
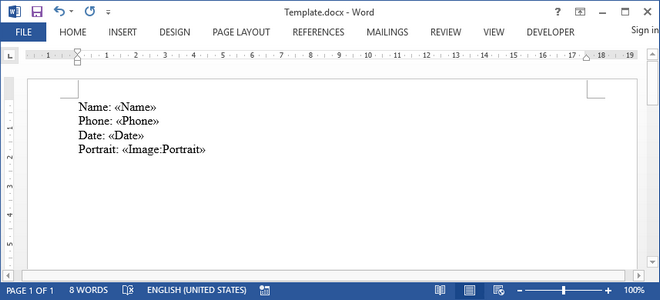
Example 2. Mail merge text
import com.spire.doc.Document;
import com.spire.doc.FileFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
public class MailMergeText {
public static void main(String[] args) throws Exception {
//Create a Document instance
Document document = new
Document();
//Load the template
document.loadFromFile("C:\\Users\\Administrator\\Desktop\\Template.docx");
//Specify mail merge fields' names
String[] filedNames = new
String[]{"Name", "Phone", "Date"};
//Specify text values for the fields
Date currentTime = new
Date();
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String dateString = formatter.format(currentTime);
String[] filedValues = new String[]{"John Smith", "+1 (69) 123456", dateString};
//Merge the specified values into fields
document.getMailMerge().execute(filedNames, filedValues);
//Save the document to file
document.saveToFile("MergeTextValue.docx", FileFormat.Docx_2013);
}
}
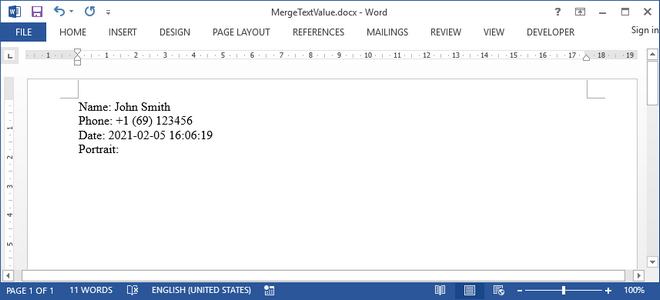
Example 3. Mail merge image
import com.spire.doc.Document;
import com.spire.doc.FileFormat;
import com.spire.doc.reporting.MergeImageFieldEventArgs;
import com.spire.doc.reporting.MergeImageFieldEventHandler;
public class MailMergeImage {
public static void main(String[] args) throws Exception {
//Create a Document object
Document document = new
Document();
//Load the template document
document.loadFromFile("C:\\Users\\Administrator\\Desktop\\Template.docx");
//Specify the field's name
String[] filedNames = new
String[]{"Portrait"};
//Specify the path of image
String[] filedValues = new
String[]{"C:\\Users\\Administrator\\Desktop\\portrait.jpg"};
//Invoke the custom method to load image
document.getMailMerge().MergeImageField = new MergeImageFieldEventHandler() {
public void invoke(Object sender, MergeImageFieldEventArgs args) {
mailMerge_MergeImageField(sender, args); //mailMerge_LoadImage
}
};
//Merge image to the field
document.getMailMerge().execute(filedNames, filedValues);
//Save the document to file
document.saveToFile("output/MergeImage.docx", FileFormat.Docx_2013);
}
//Create a custom method to load image for mail merge
private static void mailMerge_MergeImageField (Object sender,
MergeImageFieldEventArgs field) {
String filePath = field.getImageFileName();
if (filePath != null && !"".equals(filePath)) {
try {
field.setImage(filePath);
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
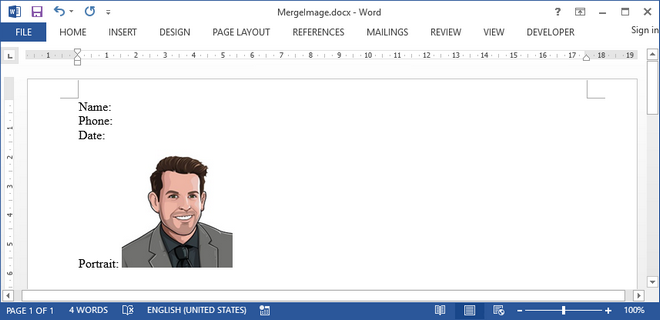
Write a comment