When splitting a PowerPoint document, you can save each slide out of the original document as a single document, or you can save some slides as one document and the rest as another document. In this article, I am going to show you how to split a PowerPoint document by using Spire.Presentation for Java.
Below is a screenshot of the sample PowerPoint document.
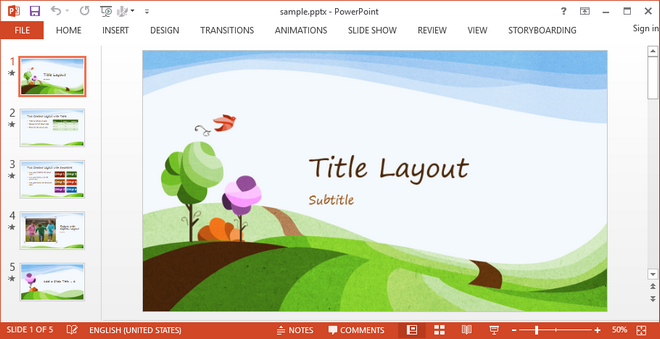
Installing Spire.Presentation.jar
If you create a Maven project, you can easily import the jar in your application using the following configurations. For non-Maven projects, download the jar file from this link and add it as a dependency in your application.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>http://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId> e-iceblue </groupId>
<artifactId>spire.presentation</artifactId>
<version>3.12.4</version>
</dependency>
</dependencies>
Example 1. Split into multiple one-page documents
import com.spire.presentation.FileFormat;
import com.spire.presentation.Presentation;
public class SplitDocument {
public static void main(String[] args) throws Exception {
//Create a Presentation object
Presentation presentation = new
Presentation();
//Load a PowerPoint document
presentation.loadFromFile("C:/Users/Administrator/Desktop/sample.pptx");
//Declare a Presentation variable
Presentation newPpt;
//Loop through the slides of the source document
for (int i = 0; i < presentation.getSlides().getCount(); i++) {
//Initialize the Presentation object
newPpt = new Presentation();
//Remove the default slide
newPpt.getSlides().removeAt(0);
//Add the specific slide of the source document to the new PowerPoint
document
newPpt.getSlides().append(presentation.getSlides().get(i));
//Save to file
newPpt.saveToFile(String.format("C:/Users/Administrator/Desktop/Output/Sub-Document-%d.pptx", i + 1), FileFormat.PPTX_2013);
}
}
}
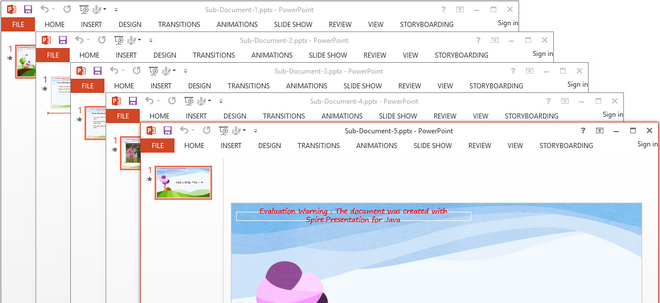
Example 2. Split by page ranges
import com.spire.presentation.FileFormat;
import com.spire.presentation.Presentation;
public class SplitByRanges {
public static void main(String[] args) throws Exception {
//Create a Presentation object
Presentation presentation = new
Presentation();
//Load a PowerPoint document
presentation.loadFromFile("C:/Users/Administrator/Desktop/sample.pptx");
//Create a new PowerPoint document
Presentation newPpt1 = new
Presentation();
//Remove the default slide
newPpt1.getSlides().removeAt(0);
//Add the slide 1 and slide 2 of the source document to the new document
for (int i = 0; i < 2; i++) {
newPpt1.getSlides().append(presentation.getSlides().get(i));
}
//Save the new document
newPpt1.saveToFile("Splited-1.pptx", FileFormat.PPTX_2013);
//Create another document to hold the remaining slides
Presentation newPpt2 = new
Presentation();
newPpt2.getSlides().removeAt(0);
for (int j = 2; j < presentation.getSlides().getCount(); j++) {
newPpt2.getSlides().append(presentation.getSlides().get(j));
}
newPpt2.saveToFile("Splited-2.pptx",
FileFormat.PPTX_2013);
}
}
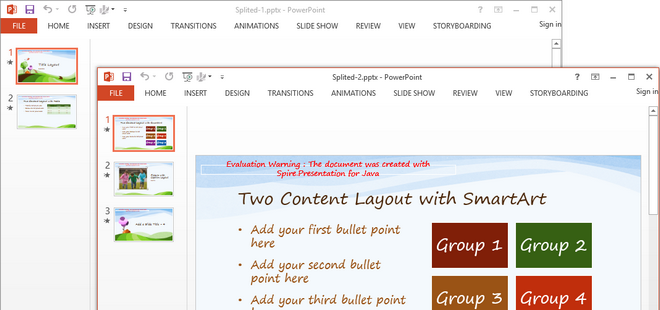
Write a comment