We often convert Excel to PDF documents in order to facilitate delivery among different devices. This article will show you how to convert an entire Excel workbook to a single PDF file and how to convert a specified worksheet to a PDF file, by using Free Spire.XLS for Java. During conversion, the cell width can be adjusted to fit the content so that the cell content can be fully displayed in the converted PDF document, and the PDF page size can be adjusted to fit content area of the sheet.
Installing Spire.Xls.jar
If you create a Maven project, you can easily import the jar in your application using the following configurations. For non-Maven projects, download the jar file from this link and add it as a dependency in your application.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>http://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId> e-iceblue </groupId>
<artifactId>spire.xls.free</artifactId>
<version>3.9.1</version>
</dependency>
</dependencies>
Example 1. Convert a workbook to PDF
import com.spire.xls.FileFormat;
import com.spire.xls.Workbook;
public class ConvertExcelToPDF {
public static void main(String[] args) {
//Create a Workbook object
Workbook workbook = new
Workbook();
//Load a sample Excel file
workbook.loadFromFile("C:\\Users\\Administrator\\Desktop\\data.xlsx");
//Make the PDF page width fit to the sheet content
workbook.getConverterSetting().setSheetFitToWidth(true);
//Autofit column width when converting
workbook.getConverterSetting().isCellAutoFit(true);
//Save to file
workbook.saveToFile("WorkbookToPDF.pdf", FileFormat.PDF);
}
}
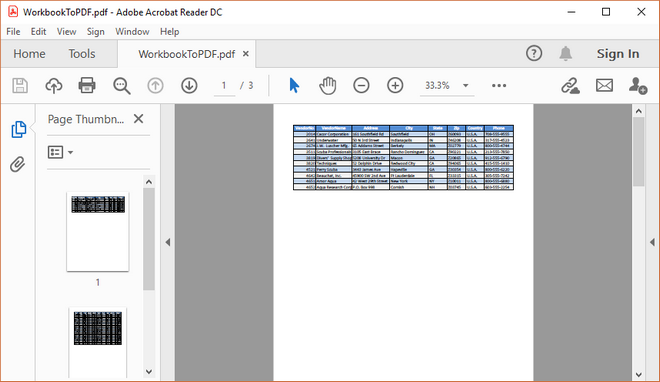
Example 2. Convert a worksheet to PDF
import com.spire.xls.Workbook;
import com.spire.xls.Worksheet;
public class ConvertSheetToPDF {
public static void main(String[] args) {
//Create a Workbook instance
Workbook workbook = new
Workbook();
//Load a sample Excel file
workbook.loadFromFile("C:\\Users\\Administrator\\Desktop\\data.xlsx");
//Make the PDF page size fit to content in a worksheet
workbook.getConverterSetting().setSheetFitToPage(true);
//Autofit column width when converting
workbook.getConverterSetting().isCellAutoFit(true);
//Get the first worksheet
Worksheet worksheet = workbook.getWorksheets().get(0);
//Convert the worksheet to PDF
worksheet.saveToPdf("SheetToPDF.pdf");
}
}
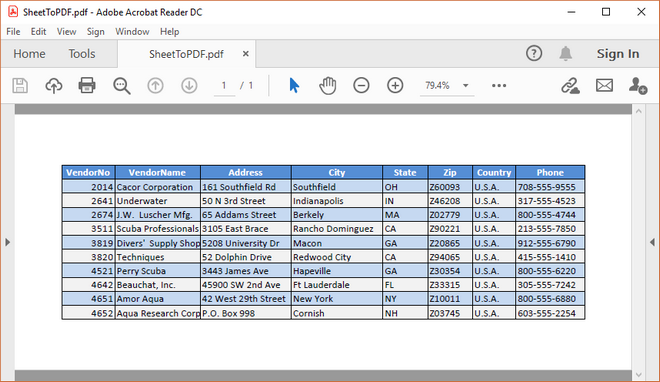
Write a comment