A watermark is a faded background image that displays behind the text in a document. It can be used to indicate a document’s status (confidential, draft, etc.) or to add a company logo. In this article, I am going to show you how to add text or image watermark to Word documents by using Free Spire.Doc for Java.
Installing Spire.Doc.jar
If you create a Maven project, you can easily import the jar in your application using the following configurations. For non-Maven projects, download the jar file from this link and add it as a dependency in your application.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>http://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId> e-iceblue </groupId>
<artifactId>spire.doc.free</artifactId>
<version>2.7.3</version>
</dependency>
</dependencies>
Example 1. Add one-line text watermark to Word
import com.spire.doc.Document;
import com.spire.doc.FileFormat;
import com.spire.doc.TextWatermark;
import com.spire.doc.documents.WatermarkLayout;
import java.awt.*;
public class InsertTextWatermark {
public static void main(String[] args) {
//Create a Document object
Document document = new Document();
//Load a Word document
document.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.docx");
//Create a TextWatermark object
TextWatermark txtWatermark = new TextWatermark();
//Set text of the watermark
txtWatermark.setText("Confidential");
txtWatermark.setFontSize(60);
txtWatermark.setColor(Color.darkGray);
txtWatermark.setLayout(WatermarkLayout.Diagonal);
//Apply the watermark to the document
document.setWatermark(txtWatermark);
//Save the document
document.saveToFile("InsertTextWatermark.docx", FileFormat.Docx );
}
}
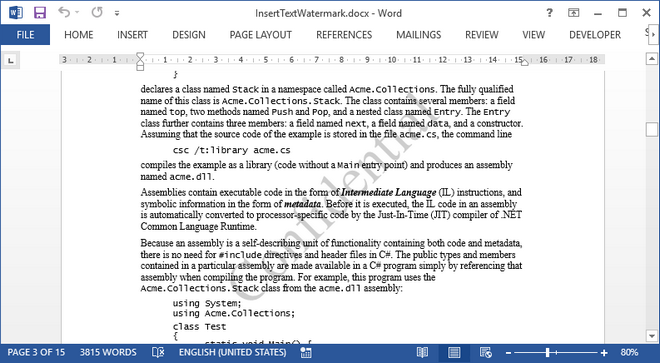
Example 2. Add multi-line text watermark to Word
import com.spire.doc.Document;
import com.spire.doc.FileFormat;
import com.spire.doc.HeaderFooter;
import com.spire.doc.Section;
import
com.spire.doc.documents.Paragraph;
import com.spire.doc.documents.ShapeLineStyle;
import com.spire.doc.documents.ShapeType;
import com.spire.doc.fields.ShapeObject;
import java.awt.*;
public class InsertMultiLineTextWatermark {
public static void main(String[] args) {
//Create a Document object
Document doc = new Document();
//Load the sample document
doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.docx");
//Create a shape
ShapeObject shape = new
ShapeObject(doc, ShapeType.Text_Plain_Text);
shape.setWidth(100);
shape.setHeight(30);
//Set the text, position and style of the shape
shape.setVerticalPosition(10);
shape.setHorizontalPosition(20);
shape.setRotation(325);
shape.getWordArt().setText("Confidential");
shape.setFillColor(Color.darkGray);
shape.setLineStyle(ShapeLineStyle.Single);
shape.setStrokeColor(new Color(192, 192, 192, 255));
//Declare two variables
Section section;
HeaderFooter header;
Paragraph paragraph;
//Loop through the sections in the document
for (int n = 0; n < doc.getSections().getCount(); n++) {
//Get the specific section
section = doc.getSections().get(n);
//Get the header of section
header = section.getHeadersFooters().getHeader();
for (int rowNum = 0; rowNum < 4; rowNum ++) {
//Add a paragraph to header
paragraph = header.addParagraph();
for (int colNum = 0; colNum < 3; colNum++) {
//Add the shape to various
positions
shape = (ShapeObject)
shape.deepClone();
shape.setVerticalPosition(50 + 150 * rowNum);
shape.setHorizontalPosition( 150 * colNum);
paragraph.getChildObjects().add(shape);
}
}
}
//Save to file
doc.saveToFile("MultiLineTextWatermark.docx", FileFormat.Docx_2013);
}
}
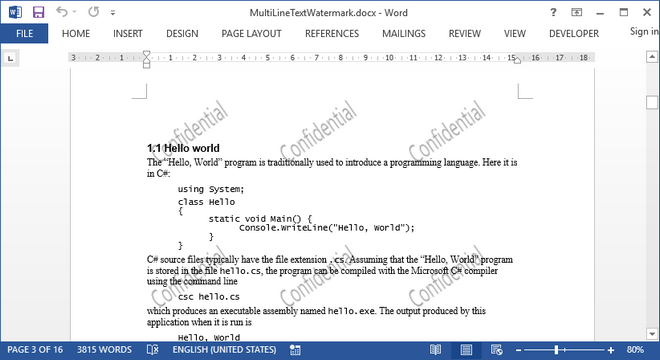
Example 3. Add an image watermark to Word
import com.spire.doc.Document;
import com.spire.doc.FileFormat;
import com.spire.doc.PictureWatermark;
public class InsertImageWatermark {
public static void main(String[] args) {
//Create a Document object
Document document = new
Document();
//Load a Word document
document.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.docx");
//Create a PictureWatermark object and set the image path
PictureWatermark picture = new
PictureWatermark();
picture.setPicture("C:\\Users\\Administrator\\Desktop\\company-logo.png");
picture.isWashout(false);
picture.setScaling(100);
//Apply image watermark to the document
document.setWatermark(picture);
//Save to file
document.saveToFile("InsertImageWatermark.docx", FileFormat.Docx );
}
}
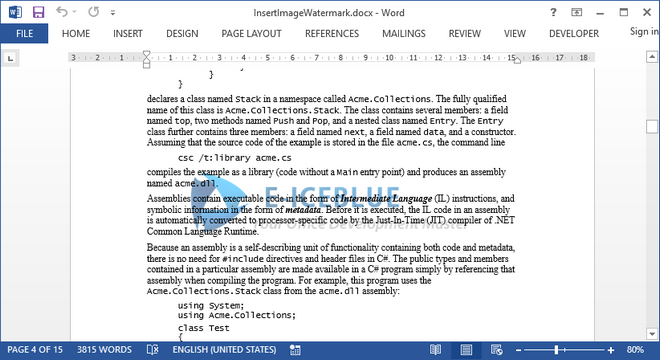
Write a comment