Creating a PowerPoint document from scratch is time-consuming, which is why there are so many pre-designed PowerPoint templates out there on the internet. In this article, I am going to show you how to replace text and images inside a PowerPoint template by using Free Spire.Presentation for Java.
Creating a template
First of all, prepare your template as follows. Make sure the text and the image are not placed within a table cell. Otherwise, the code example below won’t work. I’ll introduce how we can replace text and images within a table in my later article.
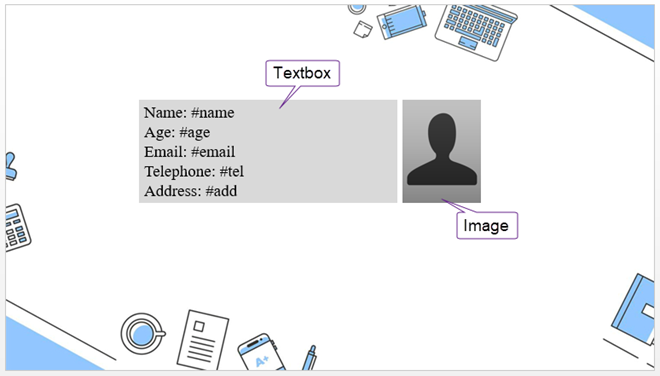
Installing Spire.Presentation.jar
If you create a Maven project, you can easily import the jar in your application using the following configurations. For non-Maven projects, download the jar file from this link and add it as a dependency in your application.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>http://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId> e-iceblue </groupId>
<artifactId>spire.presentation.free</artifactId>
<version>2.6.1</version>
</dependency>
</dependencies>
Using the code
import com.spire.presentation.*;
import com.spire.presentation.drawing.IImageData;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.FileInputStream;
import java.util.HashMap;
import
java.util.Map;
public class ReplaceTextAndImage {
public static void main(String[] args) throws Exception {
//Create a Presentation object
Presentation presentation = new
Presentation();
//Load the template file
presentation.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.pptx");
//Get the first slide
ISlide slide= presentation.getSlides().get(0);
//Create a Map object
Map<String, String> map = new HashMap<String, String>();
//Add 5 pairs of keys and values to the map
map.put("#name","Arthur Godzik");
map.put("#age","28");
map.put("#tel","863-8789");
map.put("#email","arthur1995@gmail.com");
map.put("#add","12, Sunrise Blvd, Beverly Hills, CA, US");
//Replace text in the slide
replaceText(slide,map);
//Get the image path
String picPath = "C:\\Users\\Administrator\\Desktop\\avatar.jpg";
//Replace image in the slide
replaceImage(slide, picPath);
//Save to another file
presentation.saveToFile("ReplaceTextAndImage.pptx", FileFormat.PPTX_2013);
}
public static void replaceText(ISlide slide, Map<String,
String> map) {
//Loop through the shapes in a certain slide
for (Object shape :
slide.getShapes()
) {
//Detect if the shape is an IAutoShape
if (shape instanceof IAutoShape) {
//Loop through the paragraphs in the shape
for (Object paragraph : ((IAutoShape) shape).getTextFrame().getParagraphs()
) {
ParagraphEx paragraphEx = (ParagraphEx) paragraph;
for
(String key : map.keySet()
) {
if (paragraphEx.getText().contains(key)) {
//Replace text in the paragraph
paragraphEx.setText(paragraphEx.getText().replace(key, map.get(key)));
}
}
}
}
}
}
public static void replaceImage(ISlide slide, String
picPath) throws Exception{
//Load am image from URL
BufferedImage bufferedImage = ImageIO.read(new FileInputStream(picPath));
IImageData image = slide.getPresentation().getImages().append(bufferedImage);
//Get shape collection of a slide
ShapeCollection shapes = slide.getShapes();
//Loop through shapes
for (int i = 0; i < shapes.getCount(); i++) {
//Detect if a shape is an instance of SlidePicture
if (shapes.get(i) instanceof SlidePicture) {
//Re-set the new image as the picture fill of the
shape
((SlidePicture)
shapes.get(i)).getPictureFill().getPicture().setEmbedImage(image);
}
}
}
}
Output
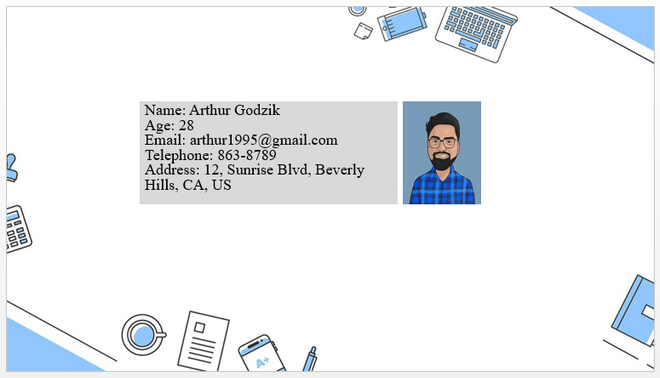
Write a comment