In this article, I’ll show you how to create a simple PDF document from scratch using Java and a free PDF library - Free Spire.PDF for Java. The PDF to be generated contains a heading, a subheading and a main body which is read from a TXT file. The font styles of these three parts are abviously different, and the headings are centrally aligned while the parahraphs of body are left aligned.
Below is a screenshot of the TXT file.
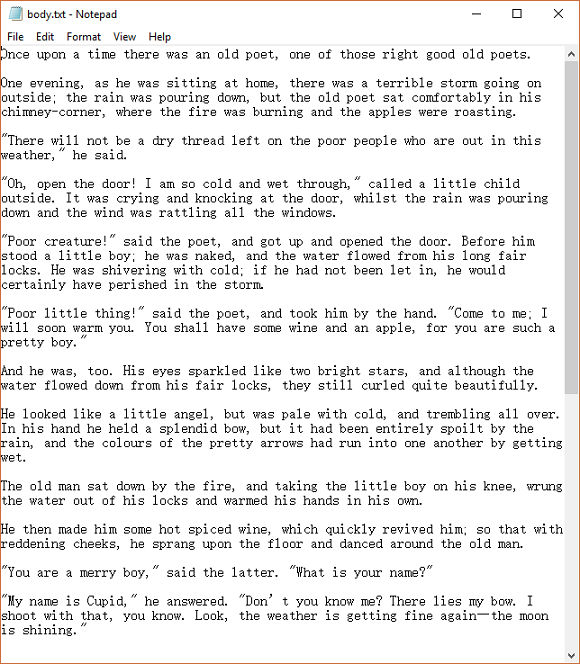
Installing Spire.Pdf.jar
If you create a Maven project, you can easily import the jar in your application using the following configurations. For non-Maven projects, download the jar file from this link and add it as a dependency in your application.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>http://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId> e-iceblue </groupId>
<artifactId>spire.pdf.free</artifactId>
<version>2.6.3</version>
</dependency>
</dependencies>
Using the code
import com.spire.pdf.graphics.*;
import java.awt.*;
import
java.awt.geom.Point2D;
import java.awt.geom.Rectangle2D;
import java.io.*;
public class CreatePdfDocument {
public static void main(String[] args) throws FileNotFoundException, IOException {
//Create a PdfDocument object
PdfDocument doc = new
PdfDocument();
//Add a page
PdfPageBase page = doc.getPages().add();
//Heading and subheading
String heading = "The Saucy
Boy";
String subheading = "by\r\nHan Christian Andersen\r\n(1835)";
//Create a red brush and a black brush
PdfSolidBrush brushBlue = new
PdfSolidBrush(new PdfRGBColor(Color.red));
PdfSolidBrush brushBlack = new PdfSolidBrush(new PdfRGBColor(Color.BLACK));
//Create three true type fonts with different style and size
PdfTrueTypeFont headingFont= new PdfTrueTypeFont(new Font("Times New Roman",Font.BOLD,20));
PdfTrueTypeFont subheadingFont= new PdfTrueTypeFont(new Font("Times New Roman",Font.BOLD,14));
PdfTrueTypeFont paraFont= new PdfTrueTypeFont(new Font("Times New Roman",Font.PLAIN,13),true);
//Set the text alignment to center via PdfStringFormat object
PdfStringFormat format1 = new
PdfStringFormat();
format1.setAlignment(PdfTextAlignment.Center);
//Draw heading and subheading on the center of the page
page.getCanvas().drawString(heading, headingFont, brushBlue, new Point2D.Float((float)page.getClientSize().getWidth()/2, 0),format1);
page.getCanvas().drawString(subheading, subheadingFont, brushBlack, new Point2D.Float((float)page.getClientSize().getWidth()/2, 30),format1);
//Get body text from a txt file
String body = readFileToString("C:\\Users\\Administrator\\Desktop\\body.txt");
//Create a PdfTextWidget object
PdfTextWidget widget = new
PdfTextWidget(body, paraFont, brushBlack);
//Create a rectangle where the body text will be drawn
Rectangle2D.Float rect = new
Rectangle2D.Float(0, 100, (float)page.getClientSize().getWidth(),(float)page.getClientSize().getHeight());
//Set the PdfLayoutType to Paginate so that the content paginate automatically
PdfTextLayout layout = new
PdfTextLayout();
layout.setLayout(PdfLayoutType.Paginate);
//Draw body text on the page
widget.draw(page, rect, layout);
//Save the document
doc.saveToFile("output/CreatePdf.pdf");
}
//Read txt file to String
private static String readFileToString(String filepath)
throws FileNotFoundException, IOException {
StringBuilder sb = new StringBuilder();
String s ="";
BufferedReader br = new BufferedReader(new FileReader(filepath));
while( (s = br.readLine()) !=
null) {
sb.append(s + "\n");
}
br.close();
String str = sb.toString();
return str;
}
}
Output-1
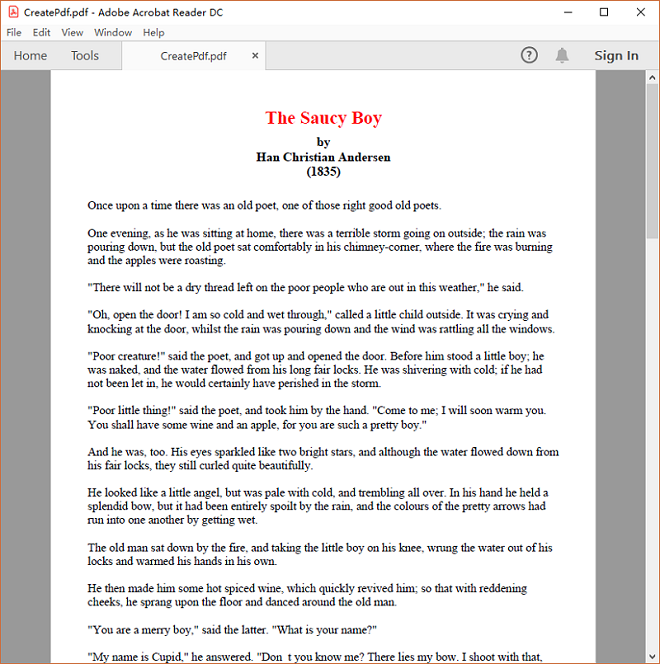
Output-2
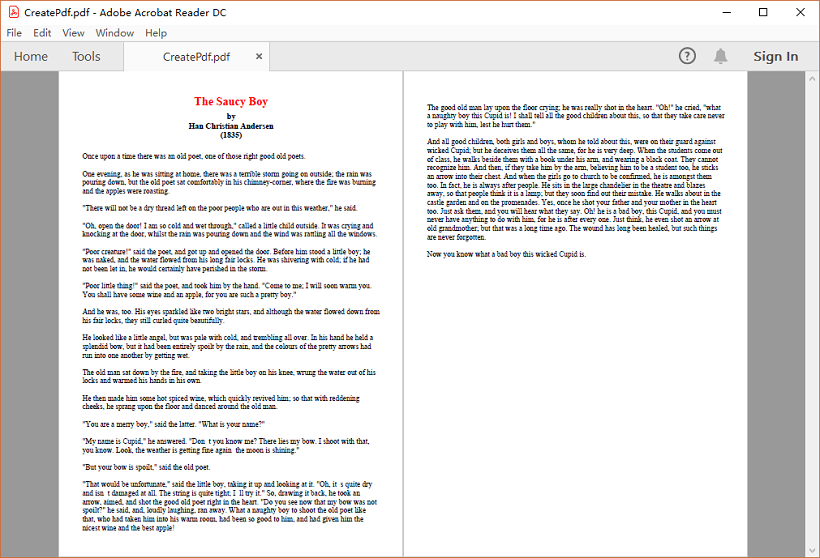
Write a comment