In this article, I’ll share with you an example of how to create a Word document, insert a few paragraphs, and how to format paragraphs as well in a Java application. This scenario depends on a Java library– Free Spire.Doc for Java, which is a free and easy-to-use library for Word documents.
Installing Spire.Doc.jar
If you create a Maven project, you can easily import the jar in your applciation using the following configurations. For non-Maven projects, please download the jar file from this link and add it as a dependency in your applicaiton.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>http://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId> e-iceblue </groupId>
<artifactId>spire.doc.free</artifactId>
<version>2.7.3</version>
</dependency>
</dependencies>
Using the code
import com.spire.doc.Document;
import com.spire.doc.FileFormat;
import com.spire.doc.Section;
import
com.spire.doc.documents.BuiltinStyle;
import com.spire.doc.documents.Paragraph;
import com.spire.doc.documents.ParagraphStyle;
import com.spire.doc.fields.TextRange;
import java.awt.*;
public class CreateWordDocument {
public static void main(String[] args) {
//Create a Word document
Document document = new
Document();
//Add a section
Section section = document.addSection();
//Add a heading
Paragraph heading = section.addParagraph();
heading.appendText("Brief Introduction of Java");
//Add a subheading
Paragraph subheading_1 = section.addParagraph();
subheading_1.appendText("What's Java");
//Add two paragraph under the first subheading
Paragraph para_1 = section.addParagraph();
para_1.appendText("Java is a general purpose, high-level programming language developed by Sun
Microsystems."+
" The Java programming language was developed by
a small team of engineers, " +
"known as the Green Team, who initiated the
language in 1991.");
Paragraph para_2 = section.addParagraph();
para_2.appendText("Originally called OAK, the Java language was designed for handheld devices and
set-top boxes. "+
"Oak was unsuccessful and in 1995 Sun changed the name to Java and modified the language to take "+
"advantage of the burgeoning World Wide Web. ");
//Add another subheading
Paragraph subheading_2 = section.addParagraph();
subheading_2.appendText("Java Today");
//Add one paragraph under the second subheading
Paragraph para_3 = section.addParagraph();
para_3.appendText("Today the Java platform is a commonly used foundation for developing and
delivering content "+
"on the web. According to Oracle, there are more than 9 million Java developers worldwide and more "+
"than 3 billion mobile phones run Java.");
//Apply builtin styles to heading and subheadings
heading.applyStyle(BuiltinStyle.Title);
subheading_1.applyStyle(BuiltinStyle.Heading_3);
subheading_2.applyStyle(BuiltinStyle.Heading_3);
//Change the font color of title paragraph
for (int i = 0; i < heading.getChildObjects().getCount(); i++) {
TextRange tr = (TextRange)heading.getChildObjects().get(i);
tr.getCharacterFormat().setTextColor(Color.BLUE);
}
//Customize a paragraph style
ParagraphStyle style = new
ParagraphStyle(document);
style.setName("paraStyle");
style.getCharacterFormat().setFontName("Arial");
style.getCharacterFormat().setFontSize(11f);
document.getStyles().add(style);
//Apply the style to other paragraphs
para_1.applyStyle("paraStyle");
para_2.applyStyle("paraStyle");
para_3.applyStyle("paraStyle");
//Set the spacing after paragraph
for (int i = 0; i < section.getParagraphs().getCount(); i++) {
section.getParagraphs().get(i).getFormat().setAfterAutoSpacing(true);
}
//Save the document
document.saveToFile("output/JavaWordDocument.docx", FileFormat.Docx);
}
}
Output
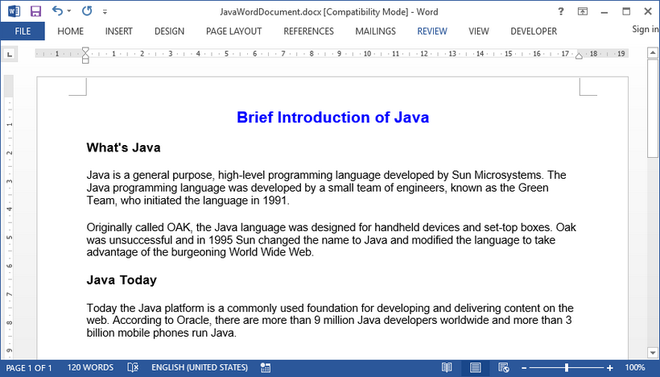
Write a comment